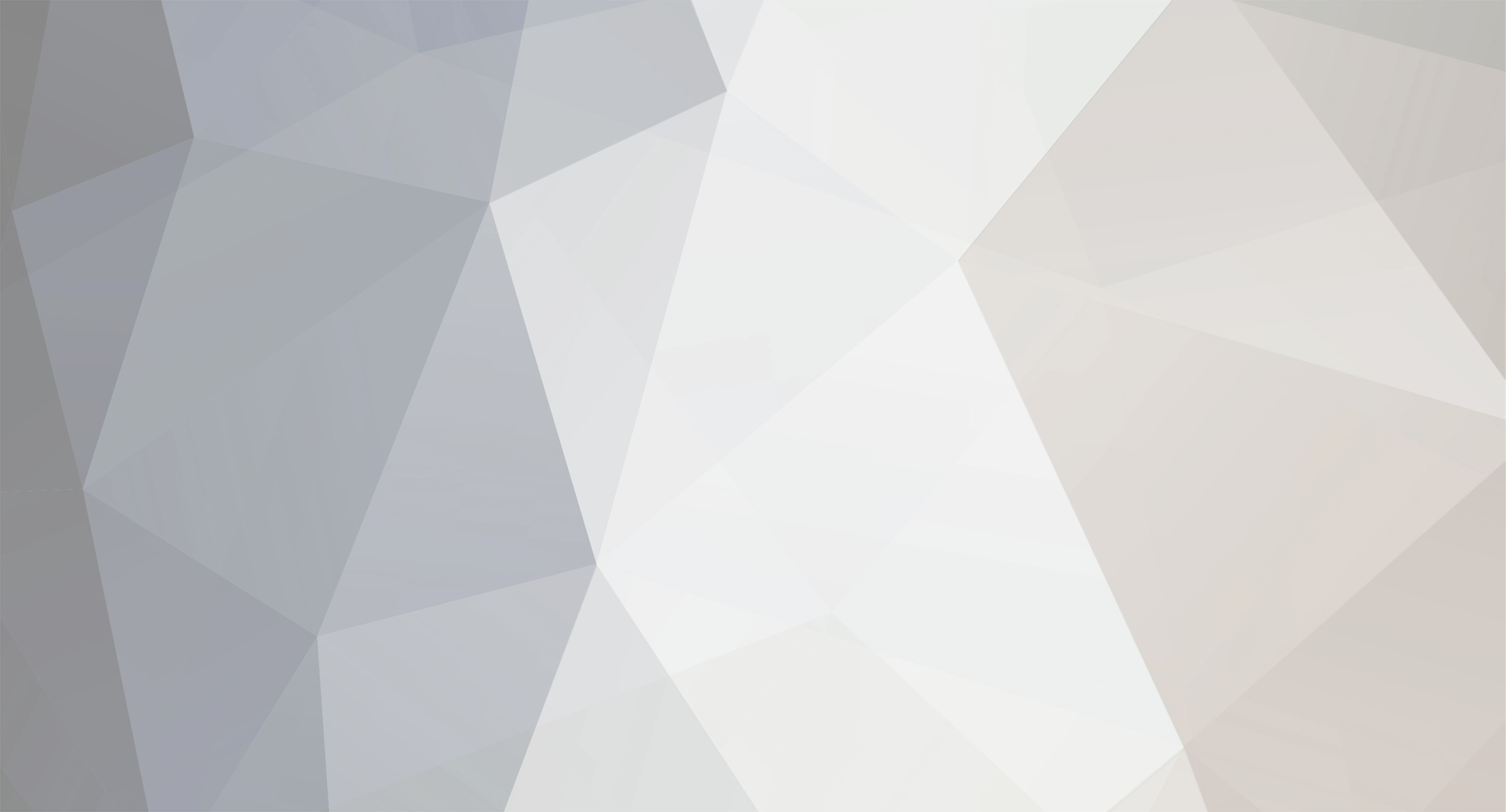
Rinart73
-
Posts
416 -
Joined
-
Last visited
-
Days Won
4
Content Type
Profiles
Forums
Events
Posts posted by Rinart73
-
-
Currently we can remove all child UI elements with the use of "UIContainer:clear()". It's good, but when building dynamic UI an ability to remove individual elements would be very handy.
-
TextBox:
It would be nice to have "setTextNoCallback" function similar to what we have with CheckBox, ComboBox and other elements.
Another great thing would be to have the "cursor" property both writable and readable. Right now if I'm changing TextBox value while user is typing, cursor is always reset to the end, which could be confusing for them.
If "cursor" was readable, I could've saved old position, changed the text and then restored the position. -
I'm pretty sure devs said already that they won't make such blocks
-
The ability to change faction name would be nice. Even if it's only when it's just being created:
function initializeAIFaction(faction) faction.name = "United Federation of Planets" -- other code end
While we do have the "Galaxy():createFaction(name, x, y)" function, it doesn't allow us to insert that faction into factionmap and automatically give it a bunch of sectors.
-
Or to add the color wheel that is already used in the Settings -> UI color
-
1
-
-
The following Player client callbacks would allow to do some build mode modding:
-
onBuildModeBlockSelected(lastBlock, blockIds) - would fire when player selects block or group of blocks.
lastBlock is the block that was just selected. blockIds represents ids of all selected blocks. -
onBuildModeBlockUnselected(lastBlock, blockIds) - would fire when player unselects block/group.
lastBlock is the id of the block that was just unselected. blockIds are all blocks that are still selected after that. Or nil/empty table if none.
Examples of that people want to do with it:
- Color-picker - I actually already have one written already
- Custom color presets/palettes
-
onBuildModeBlockSelected(lastBlock, blockIds) - would fire when player selects block or group of blocks.
-
The current bonus system is amazing. It's extremely powerful.
result = (base * baseMultiplier + multiplyableBias) * multiplier + absoluteBias
Hovewer there are still "stats" that don't follow this system:
- Thrusters - basePitch, baseRoll, baseYaw
- Entity - damageMultiplier
- Durability - maxDurabilityFactor
I understand that it would require lots of changes but I propose to change the listed stats above into StatsBonuses and to use this system for the future stats, since it allows finer control and for multiple mods to modify the same stat without conflicting each other or breaking stuff.
I've seen at least 3 separate people trying to make a yaw/roll/pitch system upgrade. They all run into issues.
I personally wanted to use all of the stats above in my mods. But currently damageMultiplier and maxDurabilityFactor are just sometimes directly set by the game which overwrites my changes. And thruster stats are reset on plan update I believe and stacking them is pain.
-
Make so ScriptUI() "registerInteraction" and "registerWindow" return their optionIndex. This will allow to properly track with what option a player interacts in following functions:
onInteract(optionIndex) onShowWindow(optionIndex) onCloseWindow(optionIndex) interactionPossible(playerIndex, optionIndex)
This is especially useful when several mods add interactions to the same entity or even modify each other.
----
On the same note it would be good to have "ScriptUI():getInteractions()" which would return a table of scripts with all their registered interactions. Numerical keys are optionIndex-es.
{ ["data/scripts/entity/merchants/researchstation.lua"] = { [0] = "Research" }, ["data/scripts/entity/merchants/consumer.lua"] = { [0] = "Trade Goods" }, ["data/scripts/entity/somemod.lua"] = { [0] = "Do this", [1] = "Do that" } }
----
Now to the matter of starting interactions via code. Currently it's only possible to start/force Dialog, but not function/window. To fix this I propose adding "interactScript" function, which would start any type of interaction in any script as long as correct script path optionIndex is provided.
ScriptUI():interactScript(scriptPath, optionIndex) -- Example: ScriptUI():interactScript("data/scripts/entity/merchants/researchstation.lua", 0)
-
Add "onClosed" callback to all "Window" objects.
Right now when I'm working with nested windows I have to hide native close button and use a fake one in order to track when user closes the window.
-
I suggest to add an explicit non-async syncronization step that would happen after client downloaded the mods and connected to a server but before any sector/entity/player scripts are created and initialized. Mods would use this step to syncronize their server-side mod configs with connecting clients and then they could use the "setGlobal" function to store them on client.
Why do we need it
Because there are cases where the standard post-initialize 'client uses invokeServerFunction("sendConfig") -> server uses invokeClientFunction("receiveConfig", config)' method doesn't work, because it's asyncronous and too late (happens after "initialize").
Examples
- Adjust item/upgrade prices - by the time we syncronize settings Equipment Docks have already initialized items with vanilla prices.
- Adjust system upgrade stats - by the time we syncronize settings, game has already created tooltips with vanilla values being shown.
- Override SectorSpecifics - imagine someone created a quest that turns volcanic planet into an earth-like planet as a result. It's an actual visible impact that player could make on a galaxy. But if someone would log in while in that sector they would still see a volcanic planet because the game requested SectorSpecifics before syncronization completed.
- And in general modifying anything that client Lua or C++ side uses during the "initialize" or before that.
As an additional bonus this will slightly decrease traffic and client RAM usage in the heavilly moded games - because some mods would syncronize configs only once (instead of once per every modded entity) and store them in one place.
How I see it
We could use something like the main.lua, but for both client and server.
Server:
SomeHelperLibrary = include("myhelperlibrary") function synchronize() local config = SomeHelperLibrary.loadConfig("ModName") -- getting config ready to be sent (perhaps stripping unnecessary info) return config -- THIS will be sent to the client end
Client:
function synchronize(config) -- we receive config and save it setGlobal("MyMod", config) end
then showing updated price ("data/scripts/items/energysuppressor.lua"):
local myMod_create = create function create(item, rarity) local item = myMod_create(item, rarity) local config = getGlobal("MyMod") item.price = config.energySuppressorPrice return item end
-
I suggest posting a bug report via ingame "Report Bug" button
-
1
-
-
symbiote, Please post your client & server logs
-
There is no quick way to do this via UI.
You could try to make a backup of "C:\Users\YourProfileName\AppData\Roaming\Avorion\mods\modconfig.lua" and switch it depending on what mod set you need
-
No idea tbh, try to ask in the Discord.
You could try to ask server owner for mods list and check your subscriptions - maybe you have installed it already?
Also check your client logs for errors.
-
2 hours ago, lovepack said:
build in a zone with no NPC stations(consumer) as those might cause this conflict
Yes, they could "steal" some traders.
2 hours ago, lovepack said:though none are set to buy
If you disabled buying then yes, traders for them simply won't spawn.
2 hours ago, lovepack said:Also for the slider bar -20% to 20% is that what determines the likihood of the type of trader when this 90 second call is made?
If I remember correctly, if you have > 0% extra price, it will make traders more likely (but not guarantee) to sell goods to your factory rather than buy (because you pay extra for those goods).
And if you set < 0% price, they're more likely to buy from factory rather than sell, because you're selling goods for a cheaper price.-
1
-
-
Not really. There are 2 separate trader "streams":
- The factory-only stream that works when in the factory settings you check "actively buy/sell" goods. This will cause factory to try to "call" for traders every 90 seconds.
- The general stream that works for all consumers and factories. Every minute it chooses one of them. So big amounts of consumers/factories in one sector will kinda conflict with each other. But with reasonable amounts it's gonna be fine.
-
1
-
Quote
especially with no way to preview what you're going to submit.
There is a "Preview" button, it's the end of the formatting buttons list.
I only miss the "Spoiler" tag and old links.
-
Quote
But without being able to disable that tab i don't see a way as a modder to disable fighter production.
Tbh disabling the tab will only disable the UI - client-side part of it. A person with coding experience will find a way to bypass it.
How about using the following code? It should delete all squads blueprints on server side from time to time.
function DisableFighterProduction.getUpdateInterval() return 20 end function DisableFighterProduction.updateServer() local entity = Entity() if entity:hasComponent(ComponentType.Hangar) then local hangar = Hangar() for i = 0, hangar.numSquads then hangar:setBlueprint(i, nil) end end end
-
I think this only happens if an active tab was created on the C++ side (like the inventory tab).
You could try to use tab callbacks for your tabs to know when they're active:
local myTab = shipWindow:createTab("My Tab Name"%_t, "path/to/icon", "My Tab Name"%_t) myTab.onSelectedFunction = "onMyTabSelected" myTab.onShowFunction = "onMyTabShown"
-
Or just make Salvage & Repair mode fighters fly like the Mine mode.
-
1
-
-
Honestly it would be easier to just disable guest posts. Because Google Captcha doesn't work anymore
-
We can change sector name on the server-side:
Sector().name = "Test"
But we can't access it on the client-side. Please add readable "Sector().name" property.
-
While this is a totally wild guess, it won't hurt to try the following:
Edit server.ini: find the "[Networking]" section and inside of it find and set "sendStatsToAdmins" to "false" and check if it solves the problem.
-
No. Rifts are created via Lua script that uses galaxy seed to create random rifts. The only thing to remove rift is to change that file.
2.2 overridden native Lua function "table.concat"
in Bugs
Posted
2.2 overriden native Lua function
table.concat
:Avorion\data\scripts\lib\utility.lua
I ask you to change the function name because native
table.concat
( http://lua-users.org/wiki/TableLibraryTutorial ) works in a completely different way. Your change breaks all mods that use this function and also confuses future modders who will expect native functions to behave in a same way.